While working on one of my projects, adding text behind moving objects using Python, I wanted to make the text glow in the video. Unfortunately, I could not figure out how to create that glow effect for the text.
However, now I have a trick that can be used to produce a neon glow effect on text by using the PIL library in Python.
Let me show you how we can add a glowing text string to an image. The code and other material for this tutorial can be downloaded from here.
Load background image
First, we will need an image that will be used as the background on top of which the text will be added. I will be resizing the image to 512 x 512 pixels. You may use any size of your choice.
from PIL import Image, ImageFont, ImageDraw
# load image
colored_bg = Image.open("input_image.jpg").resize((512,512)).convert("RGBA")
# display image
colored_bg

As you can see, we have loaded the image in “RGBA” mode. R-G-B represents the red, green, and blue channels of the image. “A” is the alpha channel of the image that controls the transparency of the image. Its values range from 0 (max transparency) to 255 (no transparency).
Specify font parameters
Now let’s define a few parameters related to the font of the text. Make sure you have the ArianaVioleta-dz2K.ttf font style file in your working directory.
# Choose a font and size for the text
font_path = "ArianaVioleta-dz2K.ttf" # replace with the path to your font file
font_size = 80 # replace with your desired font size
font = ImageFont.truetype(font_path, font_size)
Create a frame for text
Next, we will create a new image or frame on which the text will be added.
text_image = Image.new('RGBA', colored_bg.size, (255, 255, 255, 0))
draw = ImageDraw.Draw(text_image)
Define text position
I will keep the text at the center of the image. You can keep the text anywhere in the image as per your requirement.
# find height and width of text
_, _, text_width, text_height = draw.textbbox(xy = (0,0), text=text, font=font)
# find starting coordinates of the text position
text_x = (text_image.width - text_width) / 2
text_y = (text_image.height - text_height) / 2
Add glow effect to text
To make the text glow, I will use multiple layers of text frames and stack them up together.
The stroke width of the font will increase frame by frame. The transparency of the text will also increase with the stroke width.
This trick will give the text a neon glow effect.
text = "Glow Effect"
# transparency values of text frames
transparency_values = [255, 80, 70, 60, 50, 40, 30, 20, 10]
for i in range(len(transparency_values)):
# Create drawing context
draw = ImageDraw.Draw(text_image)
# Add text to the new blank image
draw.text((text_x, text_y), text, (255, 255, 255, transparency_values[i]),
font=font, stroke_width=i+1)
colored_bg = Image.alpha_composite(colored_bg, text_image)
text_image = Image.new('RGBA', colored_bg.size, (255, 255, 255, 0))
# display image
colored_bg
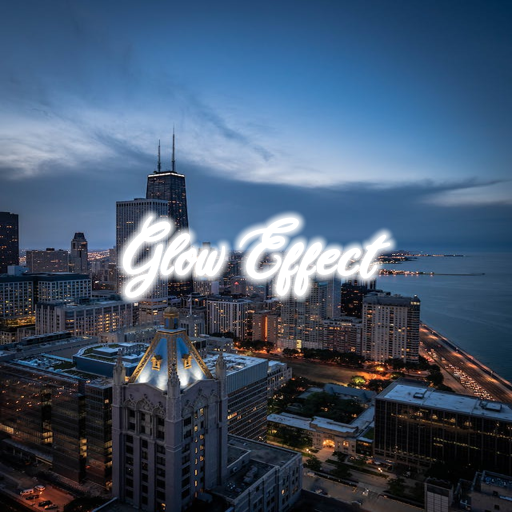
Change the color of the glowing text
We can easily change the color of the text along with its glow. All we have to do is to change the values of RGB channels inside draw.text()
function. I will use (255, 150, 100) as the new RGB values.
# load image
colored_bg = Image.open("input_image.jpg").resize((512,512)).convert("RGBA")
text_image = Image.new('RGBA', colored_bg.size, (255, 255, 255, 0))
draw = ImageDraw.Draw(text_image)
transparency_values = [255, 80, 70, 60, 50, 40, 30, 20, 10]
for i in range(len(transparency_values)):
# Create drawing context
draw = ImageDraw.Draw(text_image)
# Add text to the new blank image
draw.text((text_x, text_y), text, (255, 150, 100, transparency_values[i]),
font=font, stroke_width=i+1)
colored_bg = Image.alpha_composite(colored_bg, text_image)
text_image = Image.new('RGBA', colored_bg.size, (255, 255, 255, 0))
# Display image
colored_bg
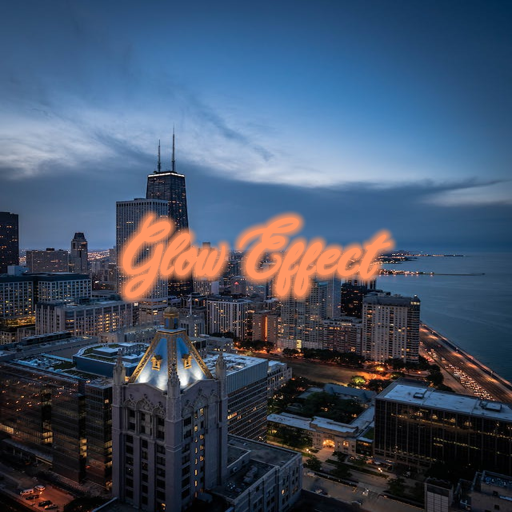
The text in the output above doesn’t seem to be glowing. In this case, we can add another layer of the same text with white color.
# Create drawing context
draw = ImageDraw.Draw(colored_bg)
# Add text to the new blank image
draw.text((text_x, text_y), text, font=font, fill=(255, 255, 255))
colored_bg

As you can see, now the text looks much better and the glowing effect is also quite visible.
The same technique can be used for videos as well if you want to add glowing text in your videos using Python.
Feel free to download the Python code, image, and font file from this link.