Scrolling text in a video or gif can add lots of value to the content and present key information in a small amount of space. To create our scrolling text animation video, we’ll use OpenCV (cv2) paired with the Python Imaging Library (PIL) in this article. Our code will also allow the users to adjust the speed of the scrolling text and also the direction of movement of the text. Ready to get started creating scrolling text animation with Python? Let’s start with right-to-left text.
Python function for creating scrolling text video
Below is a Python function that takes in inputs like a text string, speed of animation, direction of scrolling animation and a few other parameters to create a video of scrolling text either left-to-right or right-to-left.
Inside the function, we have set the width and height of the video to 300 and 200 pixels, respectively. The video background color has been set to black (0,0,0) and the color of the text is going to be white (255, 255, 255). The font that we are using for the text is Arial font, so make sure you have the arial.tff file in the working directory. Feel free to change these parameters as per your requirement.
def create_scrolling_text_video(text, font_path, font_size, speed, output_file, direction='left'):
image_width=300
image_height=200
text_color=(255, 255, 255) # scrolling text color
bg_color=(0, 0, 0) # background color
font = ImageFont.truetype(font_path, font_size)
# get size of the input text string
text_width, text_height = font.getsize(text)
# number of frames
num_frames = text_width + image_width
fourcc = cv2.VideoWriter_fourcc(*"mp4v")
video_writer = cv2.VideoWriter(output_file, fourcc, speed, (image_width, image_height))
# create frames for text scrolling
for i in range(num_frames):
img = Image.new("RGB", (image_width, image_height), bg_color)
draw = ImageDraw.Draw(img)
if direction == 'left':
x = image_width - i
elif direction == 'right':
x = -(image_width - i)
else:
raise ValueError("Invalid direction. Choose either 'left' or 'right'.")
y = (image_height - text_height) // 2
draw.text((x, y), text, font=font, fill=text_color)
# Convert the image to an OpenCV frame
frame = cv2.cvtColor(np.array(img), cv2.COLOR_RGB2BGR)
video_writer.write(frame)
video_writer.release()
The number of frames has been set equal to the sum of the frame width and the input text string width. This will allow the entire text string to pass the frame from first character till the last character.
Then on each frame, at the center, the text is overlayed. After that all the frames are combined to create an mp4 video and then it is saved at the specified location in your system.
Horizontal scrolling text animation: right-to-left
Now we will call the above function to create a short video of scrolling text from right-to-left. The input text string is “This is a scrolling text animation example”.
text = "This is a scrolling text animation example."
font_path = "arial.ttf" # Path to your desired font file (e.g., arial.ttf or path to any other TTF font file)
font_size = 32
speed = 90 # Higher value makes the text scroll faster
output_file = "scrolling_text.mp4"
create_scrolling_text_video(text, font_path, font_size, speed, output_file, direction = "left")

Horizontal scrolling text animation: left-to-right
Let’s change the direction of the scrolling text and make it left-to-right. All we have to do is pass ‘left’ as the value for the ‘direction’ argument.
text = "This is a scrolling text animation example."
font_path = "arial.ttf" # Path to your desired font file (e.g., arial.ttf or path to any other TTF font file)
font_size = 26
speed = 90 # Higher value makes the text scroll faster
output_file = "scrolling_text_right.mp4"
create_scrolling_text_video(text, font_path, font_size, speed, output_file, direction = "right")
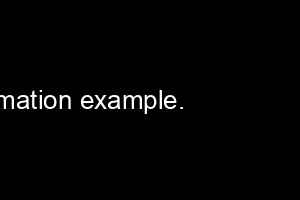
Horizontal scrolling text animation: both directions
Now let’s modify the scrolling text animation function and implement dual text scrolling animation from both the directions.
# scrolling text from both directions
def create_dual_direction_scrolling_text_video(text1, text2, font_path, font_size, speed, output_file):
image_width=200
image_height=200
text_color=(255, 255, 255)
bg_color=(0, 0, 0)
font = ImageFont.truetype(font_path, font_size)
text1_width, text1_height = font.getsize(text1)
text2_width, text2_height = font.getsize(text2)
num_frames = max(text1_width, text2_width) + image_width
fourcc = cv2.VideoWriter_fourcc(*"mp4v")
video_writer = cv2.VideoWriter(output_file, fourcc, speed, (image_width, image_height))
for i in range(num_frames):
img = Image.new("RGB", (image_width, image_height), bg_color)
draw = ImageDraw.Draw(img)
x1 = image_width - i
y1 = (image_height - text1_height) // 3
x2 = -(image_width - i)
y2 = y1 + text2_height + 20
draw.text((x1, y1), text1, font=font, fill=text_color)
draw.text((x2, y2), text2, font=font, fill=text_color)
frame = cv2.cvtColor(np.array(img), cv2.COLOR_RGB2BGR) # Convert the image to an OpenCV frame
video_writer.write(frame)
video_writer.release()
Once the function is ready, we will pass some inputs to this function to generate the video of two text strings scrolling horizontally from opposite directions.
text1 = "This is scrolling text 1."
text2 = "This is scrolling text 2."
font_path = "arial.ttf" # Path to your desired font file (e.g., arial.ttf or path to any other TTF font file)
font_size = 32
speed = 30 # Higher value makes the text scroll faster
output_file = "dual_scrolling_text.mp4"
create_dual_direction_scrolling_text_video(text1, text2, font_path, font_size, speed, output_file)

As you can see, we have two text strings moving in opposite directions. Similarly, not just text, we can also make other items like icons or stickers move or scroll like this over a static background. You can easily use scrolling text in YouTube Shorts, Instagram Reels or any infograpic video.